Post Syndicated from Karthik Nagarajan original https://aws.amazon.com/blogs/security/how-to-monitor-the-expiration-of-saml-identity-provider-certificates-in-an-amazon-cognito-user-pool/
With Amazon Cognito user pools, you can configure third-party SAML identity providers (IdPs) so that users can log in by using the IdP credentials. The Amazon Cognito user pool manages the federation and handling of tokens returned by a configured SAML IdP. It uses the public certificate of the SAML IdP to verify the signature in the SAML assertion returned by the IdP. Public certificates have an expiry date, and an expired public certificate will result in a SAML user federation failing because it can no longer be used for signature verification. To avoid user authentication failures, you must monitor and rotate SAML public certificates before expiration.
You can configure SAML IdPs in an Amazon Cognito user pool by using a SAML metadata document or a URL that points to the metadata document. If you use the SAML metadata document option, you must manually upload the SAML metadata. If you use the URL option, Amazon Cognito downloads the metadata from the URL and automatically configures the SAML IdP. In either scenario, if you don’t rotate the SAML certificate before expiration, users can’t log in using that SAML IdP.
In this blog post, I will show you how to monitor SAML certificates that are about to expire or already expired in an Amazon Cognito user pool by using an AWS Lambda function initiated by an Amazon EventBridge rule.
Solution overview
In this section, you will learn how to configure a Lambda function that checks the validity period of the SAML IdP certificates in an Amazon Cognito user pool, logs the findings to AWS Security Hub, and sends out an Amazon Simple Notification Service (Amazon SNS) notification with the list of certificates that are about to expire or have already expired. This Lambda function is invoked by an EventBridge rule that uses a rate or cron expression and runs on a defined schedule. For example, if the rate expression is defined as 1 day, the EventBridge rule initiates the Lambda function once each day. Figure 1 shows an overview of this process.
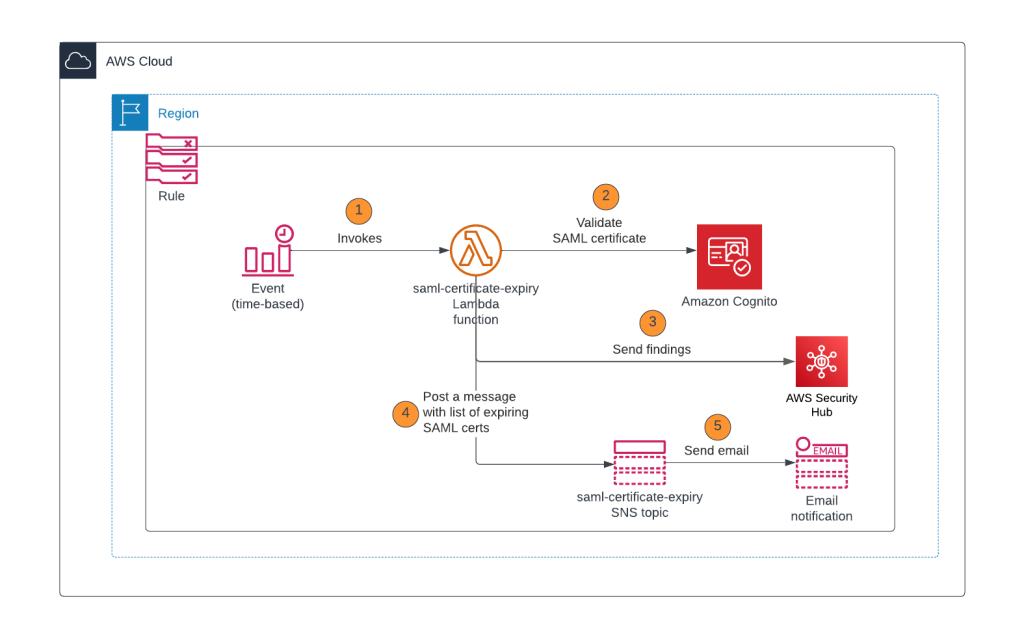
Figure 1: Lambda function initiated by EventBridge rule
As shown in Figure 1, this process involves the following steps:
- EventBridge runs a rule using a rate expression or cron expression and invokes the Lambda function.
- The Lambda function performs the following tasks:
- Gets the list of SAML IdPs and corresponding X509 certificates.
- Verifies if the X509 certificates are about to expire or already expired based on the dates in the certificate.
- Based on the results of step 2, the Lambda function logs the findings in AWS Security Hub. Each finding shows the SAML certificate that is about to expire or is already expired.
- Based on the results of step 2, the Lambda function publishes a notification to the Amazon SNS topic with the certificate expiration details. For example, if CERT_EXPIRY_DAYS=60, the details of SAML certificates that are going to expire within 60 days or are already expired are published in the SNS notification.
- Amazon SNS sends messages to the subscribers of the topic, such as an email address.
Prerequisites
For this setup, you will need to have the following in place:
- An Amazon Cognito user pool
- One or more SAML IdPs in the Amazon Cognito user pool
- Node.js 16
- AWS Security Hub enabled
Implementation details
In this section, we will walk you through how to deploy the Lambda function and configure an EventBridge rule that invokes the Lambda function.
Step 1: Create the Node.js Lambda package
- Open a command line terminal or shell.
- Create a folder named saml-certificate-expiration-monitoring.
- Install the fast-xml-parser module by running the following command:
cd saml-certificate-expiration-monitoring npm install fast-xml-parser
- Create a file named index.js and paste the following content in the file.
const AWS = require('aws-sdk'); const { X509Certificate } = require('crypto'); const { XMLParser} = require("fast-xml-parser"); const https = require('https'); exports.handler = async function(event, context, callback) { const cognitoUPID = process.env.COGNITO_UPID; const expiryDays = process.env.CERT_EXPIRY_DAYS; const snsTopic = process.env.SNS_TOPIC_ARN; const postToSh = process.env.ENABLE_SH_MONITORING; //Enable security hub monitoring var securityhub = new AWS.SecurityHub({apiVersion: '2018-10-26'}); var shParams = { Findings: [] }; AWS.config.apiVersions = { cognitoidentityserviceprovider: '2016-04-18', }; // Initialize CognitoIdentityServiceProvider. const cognitoidentityserviceprovider = new AWS.CognitoIdentityServiceProvider(); let listProvidersParams = { UserPoolId: cognitoUPID /* required */ }; let hasNext = true; const providerNames = []; while (hasNext) { const listProvidersResp = await cognitoidentityserviceprovider.listIdentityProviders(listProvidersParams).promise(); listProvidersResp['Providers'].forEach(function(provider) { if(provider.ProviderType == 'SAML') { providerNames.push(provider.ProviderName); } }); listProvidersParams.NextToken = listProvidersResp.NextToken; hasNext = !!listProvidersResp.NextToken; //Keep iterating if there are more pages } let describeIdentityProviderParams = { UserPoolId: cognitoUPID /* required */ }; //Initialize the options for fast-xml-parser //Parse KeyDescriptor as an array const alwaysArray = [ "EntityDescriptor.IDPSSODescriptor.KeyDescriptor" ]; const options = { removeNSPrefix: true, isArray: (name, jpath, isLeafNode, isAttribute) => { if( alwaysArray.indexOf(jpath) !== -1) return true; }, ignoreDeclaration: true }; const parser = new XMLParser(options); let certExpMessage = ''; const today = new Date(); if(providerNames.length == 0) { console.log("There are no SAML providers in this Cognito user pool. ID : " + cognitoUPID); } for (let provider of providerNames) { describeIdentityProviderParams.ProviderName = provider; const descProviderResp = await cognitoidentityserviceprovider.describeIdentityProvider(describeIdentityProviderParams).promise(); let xml = ''; //Read SAML metadata from Cognito if the file is available. Else, read the SAML metadata from URL if('MetadataFile' in descProviderResp.IdentityProvider.ProviderDetails) { xml = descProviderResp.IdentityProvider.ProviderDetails.MetadataFile; } else { let metadata_promise = getMetadata(descProviderResp.IdentityProvider.ProviderDetails.MetadataURL); xml = await metadata_promise; } let jObj = parser.parse(xml); if('EntityDescriptor' in jObj) { //SAML metadata can have multiple certificates for signature verification. for (let cert of jObj['EntityDescriptor']['IDPSSODescriptor']['KeyDescriptor']) { let certificate = '-----BEGIN CERTIFICATE-----\n' + cert['KeyInfo']['X509Data']['X509Certificate'] + '\n-----END CERTIFICATE-----'; let x509cert = new X509Certificate(certificate); console.log("------ Provider : " + provider + "-------"); console.log("Cert Expiry: " + x509cert.validTo); const diffTime = Math.abs(new Date(x509cert.validTo) - today); const diffDays = Math.ceil(diffTime / (1000 * 60 * 60 * 24)); console.log("Days Remaining: " + diffDays); if(diffDays <= expiryDays) { certExpMessage += 'Provider name: ' + provider + ' SAML certificate (serialnumber : '+ x509cert.serialNumber + ') expiring in ' + diffDays + ' days \n'; if(postToSh === 'true') { //Log finding for security hub logFindingToSh(context, shParams, 'Provider name: ' + provider + ' SAML certificate is expiring in ' + diffDays + ' days. Please contact the Identity provider to rotate the certificate.', x509cert.fingerprint, cognitoUPID, provider); } } } } } //Send a SNS message if a certificate is about to expire or already expired if(certExpMessage) { console.log("SAML certificates expiring within next " + expiryDays + " days :\n"); console.log(certExpMessage); certExpMessage = "SAML certificates expiring within next " + expiryDays + " days :\n" + certExpMessage; // Create publish parameters let snsParams = { Message: certExpMessage, /* required */ TopicArn: snsTopic }; // Create promise and SNS service object let publishTextPromise = await new AWS.SNS({apiVersion: '2010-03-31'}).publish(snsParams).promise(); console.log(publishTextPromise); if(postToSh === 'true') { console.log("Posting the finding to SecurityHub"); let shPromise = await securityhub.batchImportFindings(shParams).promise(); console.log("shPromise : " + JSON.stringify(shPromise)); } } else { console.log("No certificates are expiring within " + expiryDays + " days"); } }; function getMetadata(url) { return new Promise((resolve, reject) => { https.get(url, (response) => { let chunks_of_data = []; response.on('data', (fragments) => { chunks_of_data.push(fragments); }); response.on('end', () => { let response_body = Buffer.concat(chunks_of_data); resolve(response_body.toString()); }); response.on('error', (error) => { reject(error); }); }); }); } function logFindingToSh(context, shParams, remediationMsg, certFp, cognitoUPID, provider) { const accountID = context.invokedFunctionArn.split(':')[4]; const region = process.env.AWS_REGION; const sh_product_arn = `arn:aws:securityhub:${region}:${accountID}:product/${accountID}/default`; const today = new Date().toISOString(); shParams.Findings.push( { SchemaVersion: "2018-10-08", AwsAccountId: `${accountID}`, /* required */ CreatedAt: `${today}`, /* required */ UpdatedAt: `${today}`, Title: 'SAML Certificate expiration', Description: 'SAML certificate expiry', /* required */ GeneratorId: `${context.invokedFunctionArn}`, /* required */ Id: `${cognitoUPID}:${provider}:${certFp}`, /* required */ ProductArn: `${sh_product_arn}`, /* required */ Severity: { Original: '89.0', Label: 'HIGH' }, Types: [ "Software and Configuration Checks/AWS Config Analysis" ], Compliance: {Status: 'WARNING'}, Resources: [ /* required */ { Id: `${cognitoUPID}`, /* required */ Type: 'AWSCognitoUserPool', /* required */ Region: `${region}`, Details : { Other: { "IdPIdentifier" : `${provider}` } } } ], Remediation: { Recommendation: { Text: `${remediationMsg}`, Url: `https://console.aws.amazon.com/cognito/v2/idp/user-pools/${cognitoUPID}/sign-in/identity-providers/details/${provider}` } } } ); }
- To create the deployment package for a .zip file archive, you can use a built-in .zip file archive utility or other third-party zip file utility. If you are using Linux or Mac OS, run the following command.
zip -r saml-certificate-expiration-monitoring.zip .
Step 2: Create an Amazon SNS topic
- Create a standard Amazon SNS topic named saml-certificate-expiration-monitoring-topic for the Lambda function to use to send out notifications, as described in Creating an Amazon SNS topic.
- Copy the Amazon Resource Name (ARN) for Amazon SNS. Later in this post, you will use this ARN in the AWS Identity and Access Management (IAM) policy and Lambda environment variable configuration.
- After you create the Amazon SNS topic, create email subscribers to this topic.
Step 3: Configure the IAM role and policies and deploy the Lambda function
- In the IAM documentation, review the section Creating policies on the JSON tab. Then, using those instructions, use the following template to create an IAM policy named lambda-saml-certificate-expiration-monitoring-function-policy for the Lambda role to use. Replace <REGION> with your Region, <AWS-ACCT-NUMBER> with your AWS account ID, <SNS-ARN> with the Amazon SNS ARN from Step 2: Create an Amazon SNS topic, and <USER_POOL_ID> with your Amazon Cognito user pool ID that you want to monitor.
- After the policy is created, create a role for the Lambda function to use the policy, by following the instructions in Creating a role to delegate permissions to an AWS service. Choose Lambda as the service to assume the role and attach the policy lambda-saml-certificate-expiration-monitoring-function-policy that you created in step 1 of this section. Specify a role named lambda-saml-certificate-expiration-monitoring-function-role, and then create the role.
- Review the topic Create a Lambda function with the console within the Lambda documentation. Then create the Lambda function, choosing the following options:
- Under Create function, choose Author from scratch to create the function.
- For the function name, enter saml-certificate-expiration-monitoring, and for Runtime, choose Node.js 16.x.
- For Execution role, expand Change default execution role, select Use an existing role, and select the role created in step 2 of this section.
- Choose Create function to open the Designer, and upload the zip file that was created in Step 1: Create the Node.js Lambda package.
- You should see the index.js code in the Lambda console.
- After the Lambda function is created, you will need to adjust the timeout duration. Set the Lambda timeout to 10 seconds. For more information, see the timeout entry in Configuring functions in the console. If you receive a timeout error, see How do I troubleshoot Lambda function invocation timeout errors?
- If you make code changes after uploading, deploy the Lambda function.
Step 4: Create an EventBridge rule
- Follow the instructions in creating an Amazon EventBridge rule that runs on a schedule to create a rule named saml-certificate-expiration-monitoring-rule. You can use a rate expression of 24 hours to initiate the event. This rule will invoke the Lambda function once per day.
- For Select a target, choose AWS Lambda service.
- For Lambda function, select the saml-certificate-expiration-monitoring function that you deployed in Step 3: Configure the IAM role and policies and deploy the Lambda function.
Step 5: Test the Lambda function
- Open the Lambda console, select the function that you created earlier, and configure the following environment variables:
- Create an environment variable called CERT_EXPIRY_DAYS. This specifies how much lead time, in days, you want to have before the certificate expiration notification is sent.
- Create an environment variable called COGNITO_UPID. This identifies the Amazon Cognito user pool ID that needs to be monitored.
- Create an environment variable called SNS_TOPIC_ARN and set it to the Amazon SNS topic ARN from Step 2: Create an Amazon SNS topic.
- Create an environment variable called ENABLE_SH_MONITORING and set it to true or false. If you set it to true, the Lambda function will log the findings in AWS Security Hub.
- Configure a test event for the Lambda function by using the default template and name it TC1, as shown in Figure 2.
Figure 2: Create a Lambda test case
- Run the TC1 test case to test the Lambda function. To make sure that the Lambda function ran successfully, check the Amazon CloudWatch logs. You should see the console log messages from the Lambda function. If ENABLE_SH_MONITORING is set to true in the Lambda environment variables, you will see a list of findings in AWS Security Hub for certificates with an expiry of less than or equal to the value of the CERT_EXPIRY_DAYS environment variable. Also, an email will be sent to each subscriber of the Amazon SNS topic.
Cleanup
To avoid future charges, delete the following resources used in this post (if you don’t need them) and disable AWS Security Hub.
- Lambda function
- EventBridge rule
- CloudWatch logs associated with the Lambda function
- Amazon SNS topic
- IAM role and policy that you created for the Lambda function
Conclusion
An Amazon Cognito user pool with hundreds of SAML IdPs can be challenging to monitor. If a SAML IdP certificate expires, users can’t log in using that SAML IdP. This post provides the steps to monitor your SAML IdP certificates and send an alert to Amazon Cognito user pool administrators when a certificate is about to expire so that you can proactively work with your SAML IdP administrator to rotate the certificate. Now that you’ve learned the benefits of monitoring your IdP certificates for expiration, I recommend that you implement these, or similar, controls to make sure that you’re notified of these events before they occur.
If you have feedback about this post, submit comments in the Comments section below. If you have questions about this post, start a new thread on the Amazon Cognito re:Post or contact AWS Support.
Want more AWS Security news? Follow us on Twitter.